REST vs GraphQL
Table of Contents
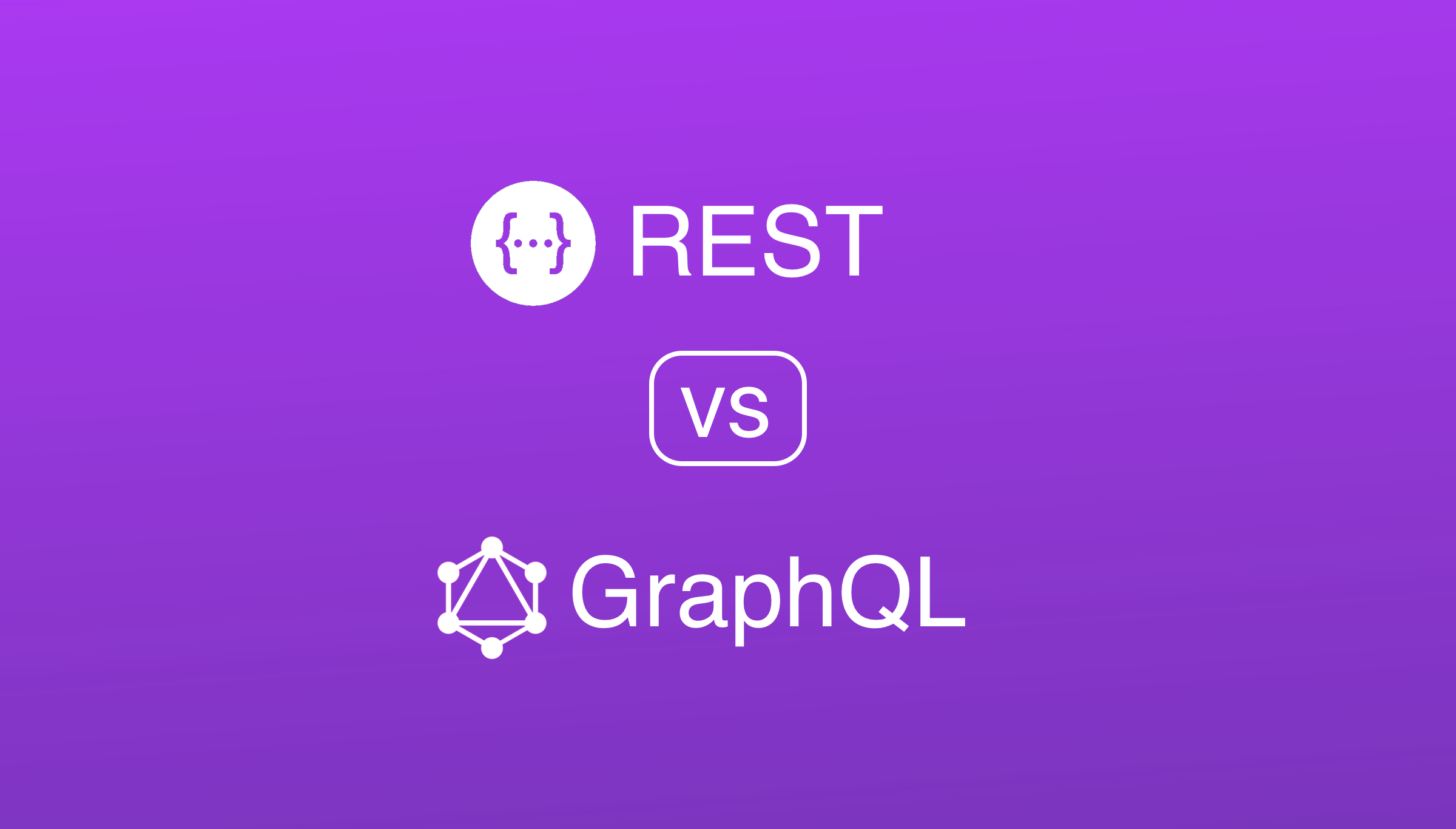
The world of web development is full of so many technologies that they cover pretty much any problem that the developer can struggle with. This number has created so many preferences and approaches toward a solution that it pretty much feels like every technology has a certain alternative for it. Nowadays we can even alter core architectural concepts like REST API and let’s discuss one such alternative called GraphQL.
What’s GraphQL? #
So what is GraphQL? Well, according to the creators of GraphQL (GraphQL Foundation (yes, these guys have no imagination)), GraphQL is a query language for APIs and a runtime for fulfilling those queries with your existing data.
So what do those heavy words mean? Let’s break that down.
When creating a full-stack application, you usually have backend and frontend teams. Backend team works on the request and response of API data structures that are later on utilized by the frontend team in some format. Those data structures are received by sending a request via a specific endpoint with specific parameters and getting specific results. However during the development cycle of the application request parameters change, response structures change, and new features are added that might need data from 2 different requests. That requires time, since frontend needs to wait for backend team and backend team, needs to stick to updating data structures.
That’s when GraphQL kicks in, allowing both teams to concentrate specifically on the functional part of the app, rather than updating data structures. It allows frontend developers to write their schemas, which means that they can get desired data without relying on defined by backend team response structures and solving the issue by sending multiple requests to fetch all the necessary data.
GraphQL vs REST #
Now let’s finally get to the comparison of GraphQL vs REST:
- GraphQL is client-driven, which means that the client decides what data to fetch, while REST is server-driven, which means that you get a specific format and specific data by sending a request to the server.
- To decide what data to send to the client, GraphQL requires a client to write a schema of the response that the client needs, while REST requires a request to a certain endpoint and by following that endpoint, you get specific data. Think about REST endpoints as a library full of books, if you need certain info, you need to ask for a book that contains that info.
- Both REST and GraphQL work over the HTTP and both of them have endpoints, unlike REST which can have lots of endpoints to access some specific data, GraphQL has only 1 endpoint with POST method, while the schema is sent as a request body. The example below demonstrates what GraphQL request body looks like:
# GraphQL request body
query {
authors {
fullName
}
}
- The popularity of REST is significantly larger than that of GraphQL, REST has been introduced back in the year 2000, while GraphQL appeared at the end of 2015, this huge gap has created a solid standard for using REST, while GraphQL kind of fades in the shadow of its’ big brother. This also impacts the community, which in the case of GraphQL is slowly but steadily growing. Below you can see the graph of trends comparison for 2022:
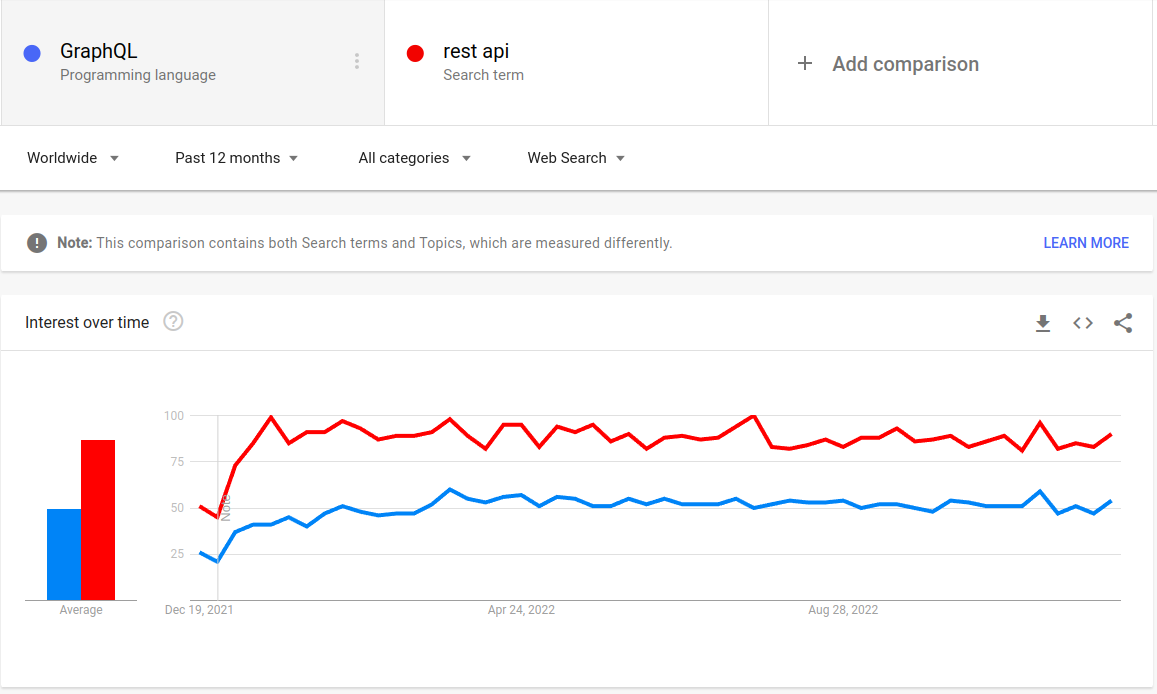
- GraphQL is easy on a surface level, however, it’s very hard to master, unlike REST, which you learn in parallel while studying web development, you might not even know what REST is and use it on daily basis.
- GraphQL documents your schemas as you write them, while REST requires you to write documentation for your endpoints on your own (yeah, there is no rest for wicked with REST). With the usage of GraphiQL (a built-in IDE for working with GraphQL that comes with the GraphQL library), you can check the correctness of the fields as you write them with no possible errors. Below, you can see documentation generated by GraphQL and displayed by GraphiQL:
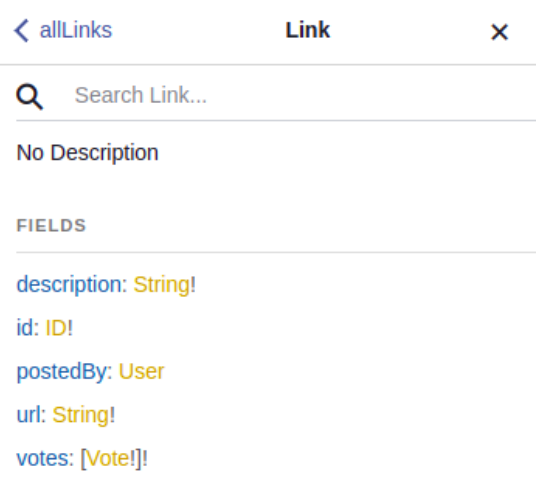
- You can’t use something like a file uploader with GraphQL, so for that, you’d need some sort of extension or a library, while REST can do anything from the get-go.
- GraphQL has a system called types, which defines an object of schema that the client can access and it provides a built-in system of type checking and validations, while utilizing REST, you’d have to write them on your own. Below, you can see an example of a type object written in Ruby, which contains field names, types, and nullability validations:
module Types
class AuthorType < Types::BaseObject
description 'Book author' # description for documentation
field :id, ID, null: false
field :first_name, String, null: true # nullability
field :last_name, String, null: true
field :yob, Int, null: false
field :is_alive, Boolean, null: false
field :created_at, GraphQL::Types::ISO8601DateTime, null: false
end
These fields validate both request and response, which means that neither backend nor client can go wrong with sending false data.
The problems of GraphQL and REST #
Just like everything else, both REST and GraphQL are not perfect.
The problems of REST are called overfetching and underfetching.
- Overfetching is when the client gets loose data that it will never use. For example, in the image below you can see an example of a response sent by REST API. Your client needs just the name and last name assigned to the user’s account to greet the user after logging in, in response, you will get absolutely all data attached to a user. This kind of data is never utilized and it might slow down the response time from the server, which means that your application will work slower.
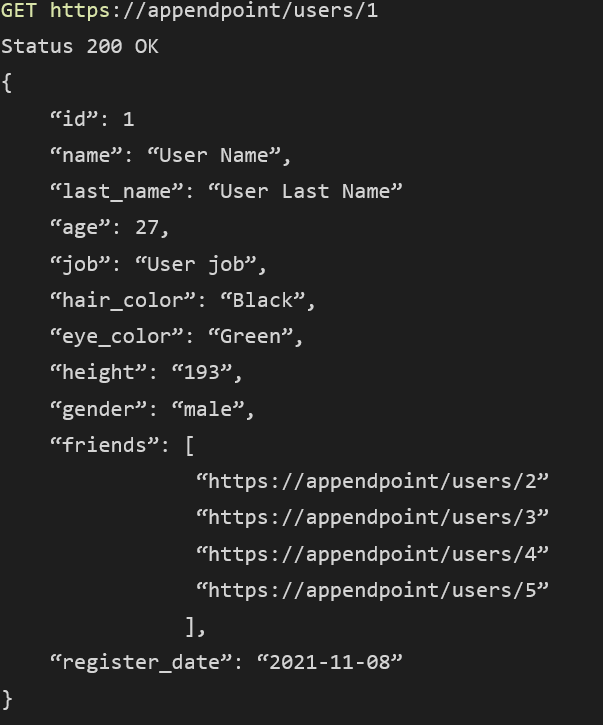
- Underfetching is the contrary issue, it means that the client doesn’t get enough data and it needs to do multiple requests. It’s an even worse problem than overfetching and in lots of case scenarios also creates overfetching. It also slows down your application, but you also trigger the server multiple times, while the server triggers the database multiple times to get you the required data, which means that in this case, 1 user acts as multiple users. Below, you can see an example of underfetching, first response gives us some data related to movies, each movie has different genres, in this case, the first response returns the ids of genres, which is the data you normally would never display to the user, instead we want to display movie’s genres, so we need to make another request to the server and fetch the genre names.
{
"page": 1,
"results": [
{
"adult": false,
"backdrop_path": "/zGLHX92Gk96O1DJvLil7ObJTbaL.jpg",
"genre_ids": [
14,
12,
28
],
"id": 338953,
"original_language": "en",
"original_title": "Fantastic Beasts: The Secrets of Dumbledore",
"overview": "Professor Albus Dumbledore knows the powerful, dark wizard Gellert Grindelwald is moving to seize control of the wizarding world. Unable to stop him alone, he entrusts magizoologist Newt Scamander to lead an intrepid team of wizards and witches. They soon encounter an array of old and new beasts as they clash with Grindelwald's growing legion of followers.",
"popularity": 195.743,
"poster_path": "/3c5GNLB4yRSLBby0trHoA1DSQxQ.jpg",
"release_date": "2022-04-06",
"title": "Fantastic Beasts: The Secrets of Dumbledore",
"video": false,
"vote_average": 6.8,
"vote_count": 3044
}],
}
}
{
"genres": [
{
"id": 12,
"name": "Adventure"
},
{
"id": 14,
"name": "Fantasy"
},
{
"id": 28,
"name": "History"
}
]
}
This is a common issue with public APIs since there are hundreds of different clients that utilize one API and you can’t satisfy the needs of all (the same issue applies to overfetching), if you have an access to the server, you can manipulate the structure of the response in such a way that satisfies the needs of the client with 1 response and to avoid the issue.
The problem with GraphQL is caching.
As we’ve already mentioned, GraphQL has only 1 endpoint, so you call only that endpoint which returns different data depending on what functionality is triggered. REST avoids this issue since it can only send a specific response by a specific endpoint, which means that data can even never be changed and always stays the same, but GraphQL having a dynamic response suffers from it, so you will always have to process the response.
GraphQL and REST together #
Despite being an alternative, it doesn’t mean that you can’t utilize GraphQL and REST together. An application can have multiple clients, it can have a webpage, software, and mobile app that utilize one server. Requests and responses utilized by a website can be different from what you want to utilize in your mobile app or if we take into account a long-running project that has passed the trial of time and is up for expansion. That’s when you might want to use both REST and GraphQL.
As we’ve already mentioned, both GraphQL and REST use HTTP for communication purposes, which means that even GraphQL has a route that it uses to communicate with the server.
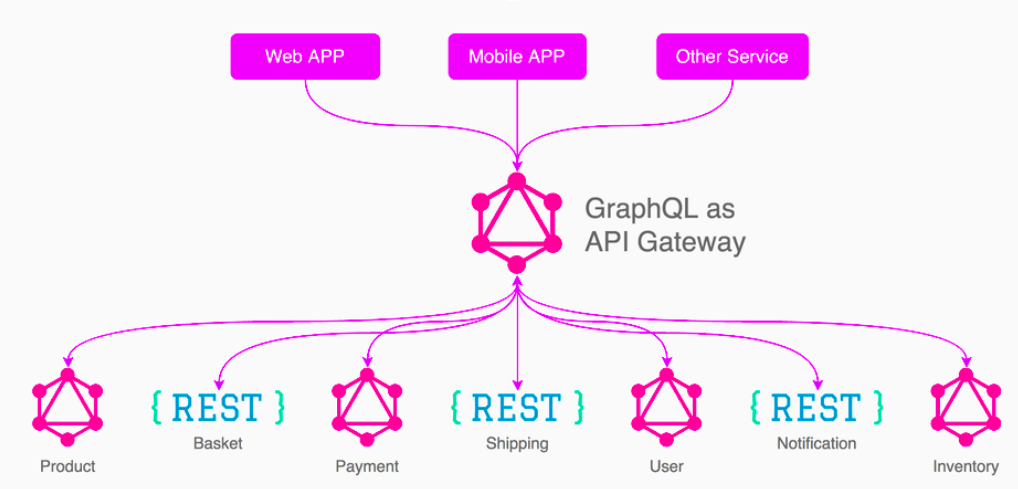
To summarize it all, GraphQL is not just an amazing alternative to REST API, but it’s also a great technology that can be used together with REST API creating solution for a large scale application for multiple platforms and public APIs. REST API is great when you have control over your server source code, while GraphQL is great when you’ve got frontend intensive apps where it’s getting hard to fixate response objects.
Now why are you still here? Go try GraphQL on your own!
We are ready to provide expert's help with your product
or build a new one from scratch for you!